Vue 3 was released in september 2020. The vue community waited for this release for a long time. During Vue Amsterdam 2019 Evan You told uw that the vue team was working on the 3rd version. But it had to be perfect. He would rather spend some more months on vue 3 and present a great version than rush it and introduce all kinds of bugs. So on Vue Amsterdam 2020 he again mentioned that the new version was almost finished. And later that year the new version was finally released. In this post I will zoom in on some interesting Vue 3 features.
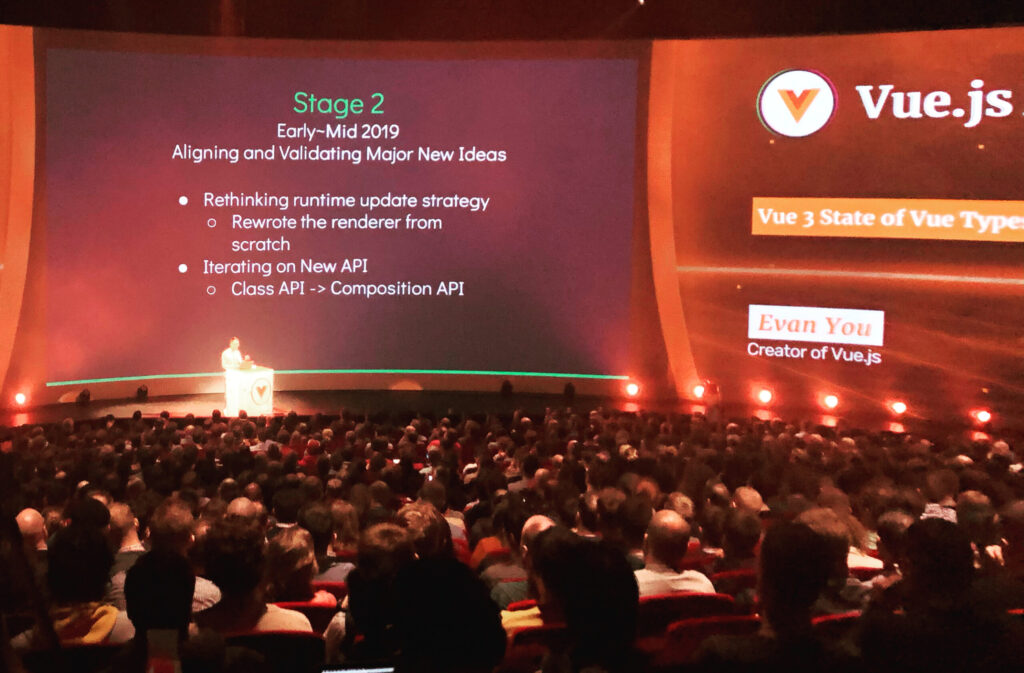
Evan You explains some new Vue 3 features.
Composition API
The composition API is basically the holy grail of Vue 3. It’s a very interesting addition that makes the life of developers a lot easier and gives us the opportunity to make our applications more dynamic.
De composition API is a way to separate template and logic in Vue. We do this by defining a setup function. In here we refer to logic so that our templates remain nice and clean. Example: I have a component in vue in which I want to display a ‘To Do list’. My template can look like this:
<template>
<div>
Add Todo:
<input type="text" v-model="todo.newTodoValue" />
<button @click="todo.addTodo()">Submit</button>
<span v-for="todoItem in todo.todoList" :key="todoItem.id">
{{ todoItem.value }}
</span>
</div>
</template>
<script>
import { useTodos } from "./hooks/todos";
export default {
name: "app",
setup() {
const todo = useTodos();
return { todo };
},
};
</script>
For logic I refer to hooks/todos which looks like this:
export const useTodos = () => {
const state = reactive({
newTodoValue: '',
todoList: [],
addTodo: function () {
let newObject = {
id: Date.now(),
value: state.newTodoValue
};
state.todoList.push(newObject);
state.newTodoValue = "";
}
});
return state;
};
export default useTodos;
This is a very basic example, and there are some arguments for keeping this in one Vue file. But I hope you get the idea. When the complexity of our application increases it becomes more and more interesting to separate template from logic. A complete working example can be found here here.
Vue 2 users can also use the composition API by including this plugin in their project. Vue 3 already has this included and no plugins are needed.
Filters are being replaced by computed properties.
Filters were a fine way to manipulate a small piece of data in our template. By appending a pipe we added a filter:
<h1>{{ message | capitalize }}</h1>
In the javascript part we add a filter:
filters: {
capitalize: function (value) {
return value.toUpperCase();
}
}
Vue 3 uses a new approach, using computed properties. So instead of a pipe we just create a new property:
<h1>{{ capitalizedmessage }}</h1>
And we define this capitalizedmessage in our computed properties:
computed: {
capitalizedmessage() {
return this.message.toUpperCase();
}
}
Computed properties are great for filtering, because it’s set up to run a query over an object very quickly. So it’s a great way to keep your front-end as performant as possible.
Little sidenote: When for example you have a lot of currencies on your screen it seems a bit complicated to create a computed property for each of those in order to format it correctly. Shaul Wildermuth wrote a nice solution for this, using a shared filter.
Teleport
Teleport is a way to move a piece of code from a component to a more generic place. Using it is really quite simple. First we create a div with a unique selector. I add this to App.vue:
<div id="modalcontainer"></div>
Whenever I want to fill this modalcontainer I simply create a ‘teleport’ element. The ‘to’ property refers to my modalcontainer in App.vue
<teleport to="#modalcontainer">
<h1>Titel</h1>
<p>Tekst</p>
<button>Close</button>
</teleport>
This way I fill the modalcontainer with the desired content. This is great for initiating modals, or if you have an error message that needs to be displayed in the same place. No hustling with event emitters.
Keep in mind that modalcontainer needs to be placed at a high level. The vue 3 documentation recommends that you place this in the index.html of your project. Personally, I prefer touching the index.html as little as possible. So I’ll just place it on top of my App.vue. But when I define my component before I define the container for my Teleport, it will not work.
Switching to Vue 3
Everything described above and more makes vue 3 a great frontend framework for setting up your application. The only remark is that not all vue 2 libraries have a vue 3 version. It may take some time before all plugins are vue 3 compatible.
Nuxt
Nuxt is a meta framework voor Vue that makes routing, module imports and rendering a lot easier and more performant. Unfortunately, Nuxt does not have vue 3 support yet. So if you plan to migrate your nuxt application to vue 3 you will have to wait.
0 Comments